Getting something like this to work is pretty straight forward but will require a little prep work depending on the state of your form.
Let's say I have the following form:
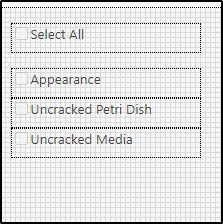
If I wanna write some code to get that Select All Yes/No Control to toggle *some* collection of other Yes/No Controls on the form, then I'll need to make sure that the Controls have names. As you can see, my controls are named after a fairly straight forward "control_SOMENAME" convention:
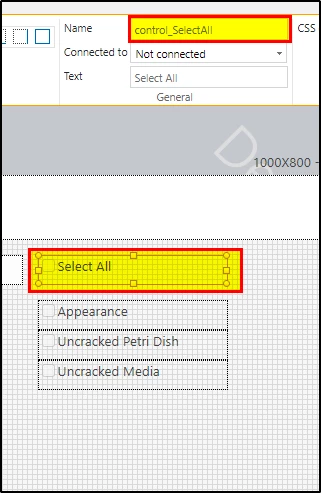
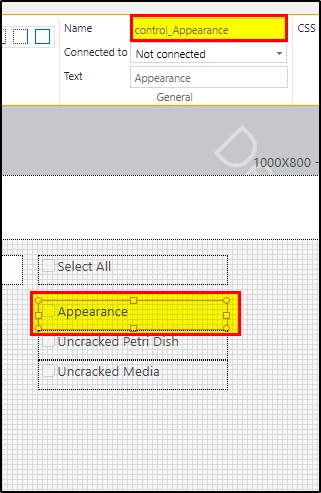
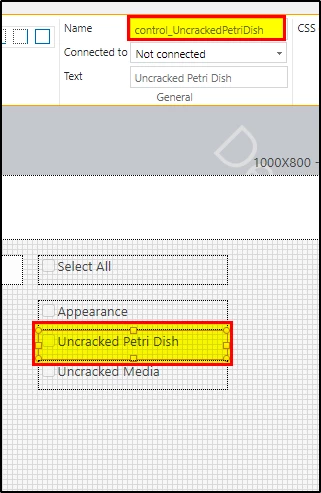
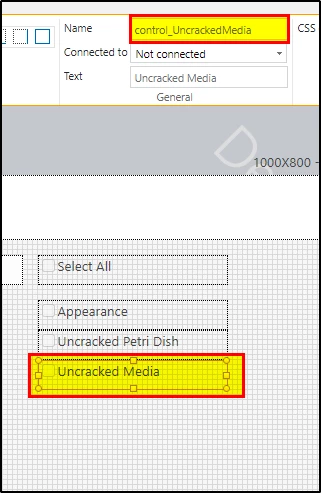
Now that I have my Controls named, I can open the Form's Settings, and Custom JavaScript dropdown:
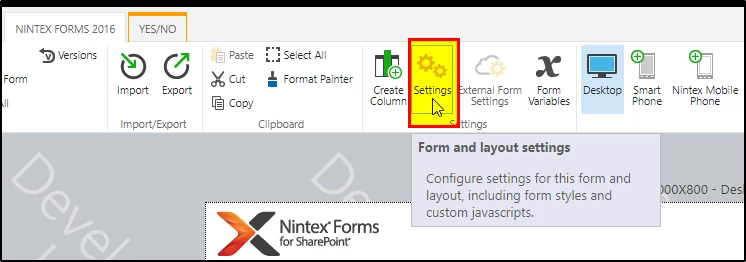
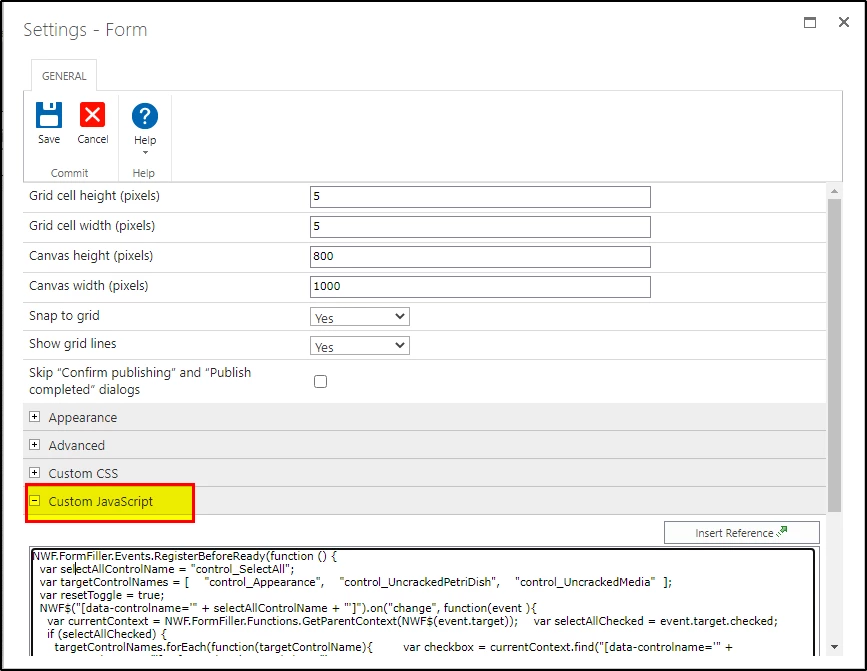
And insert the following code:
NWF.FormFiller.Events.RegisterBeforeReady(function () {
var selectAllControlName = "control_SelectAll";
var targetControlNames = [
"control_Appearance",
"control_UncrackedPetriDish",
"control_UncrackedMedia"
];
var resetToggle = true;
NWF$("[data-controlname='" + selectAllControlName + "']").on("change", function(event ){
var currentContext = NWF.FormFiller.Functions.GetParentContext(NWF$(event.target));
var selectAllChecked = event.target.checked;
if (selectAllChecked) {
targetControlNames.forEach(function(targetControlName){
var checkbox = currentContext.find("[data-controlname='" + targetControlName + "'] .nf-associated-control>input");
if (!checkbox.prop("checked")) {
checkbox.prop("checked", true).trigger("change");
}
});
if (resetToggle) {
event.target.checked = false;
}
}
});
});
Now when I preview the form I can see that when I click on the "Select All" checkbox, any unchecked boxes that I've specified will be checked:
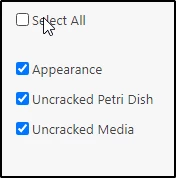
Now you may have noticed that my "Select All" is actually checked in this screenshot despite it being the thing that is controlling all of the checks below it. That's because I set it up in my above code to immediately uncheck the Select All checkbox whenever it's done checking all of the specified controls.
Luckily you can change this behavior in the code I have provided you, so let's get into how you can configure the code for your environment.
There are only Three lines of code that you should be concerned with, and those are the three main variables:
var selectAllControlName = "control_SelectAll";
var targetControlNames = [
"control_Appearance",
"control_UncrackedPetriDish",
"control_UncrackedMedia"
];
var resetToggle = true;
As I'm sure you can guess, the variable 'selectAllControlName' will need to have a value that is the Control Name of the Select All Yes/No Control for your form.
The 'targetControlNames' is an Array containing ALL of the Control Names of any Control that you'd like to be toggled by the Select All Control. In my code example I am only controlling three other Yes/No Controls, but in your case, you'll need to manually add the names of your other controls to this array!
Adding values is easy, just make sure that you're putting a comma after each entry, with the exception of the last one. Example:
var targetControlNames = [
"control_Appearance",
"control_UncrackedPetriDish",
"control_UncrackedMedia",
"control_NewControlNameHere",
"control_AnotherControlName"
];
Last but not least is the 'resetToggle' variable that controls the behavior of the SelectAll after all of the Selecting has been done. This is what clears it's checkbox. If it's set to true (as it is by default here) then when you click on "Select All" it will check all of the checkboxes, and then uncheck itself. To make it so that you need to uncheck it and then check it again to make it select everything, then simply set this to false:
var resetToggle = false;
Example of what that looks like:
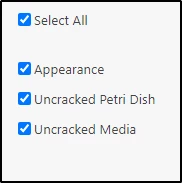
And that about does it! This code should work for controls that are inside or outside of a Repeating Section, across all rows, assuming that you've given your controls proper Control Names, and they are all typed up in their proper variable positions.
Let me know if you need further help or if you'd like an in depth explanation of the code. I would have typically included more comments, but it's incredibly late here and I'm very tired!
Good luck!
Thanks MegaJerk for the explanation and the screenshots! In this case though, the Media Quality Checks is a choice control, so it's not an individual yes/no checkbox. Should I deconstruct it into individual ones, or is there a way to reference it collectively as a whole?
I tried replacing this portion:
var targetControlNames = [
"control_Appearance",
"control_UncrackedPetriDish",
"control_UncrackedMedia",
"control_NewControlNameHere",
"control_AnotherControlName"
];
with just the mediaQualityChecks, and it didn't work.
NWF.FormFiller.Events.RegisterBeforeReady(function () {
var selectAllControlName = "checkbox_CheckAll";
var targetControlNames = [ "mediaQualityChecks" ];
var resetToggle = false;
NWF$("[data-controlname='" + selectAllControlName + "']").on("change", function(event ){
var currentContext = NWF.FormFiller.Functions.GetParentContext(NWF$(event.target)); var selectAllChecked = event.target.checked;
if (selectAllChecked) {
targetControlNames.forEach(function(targetControlName){ var checkbox = currentContext.find("[data-controlname='" + targetControlName + "'] .nf-associated-control>input");
if (!checkbox.prop("checked")) { checkbox.prop("checked", true).trigger("change"); }
});
if (resetToggle) { event.target.checked = false; } } });});
I'm assuming that since media quality checks is not an individual item, the array would fail to cycle through.
I noticed in a similar post that you also solved (haha! rad (: ) : Check All Check Boxes that the poster had used a similar inclusive checklist (list lookup checkbox control vs. my choice control) which was dependent on selection of another control.
In this case, in my form, the media quality checks is not affected by any external factor. I tried modifying the code in the following manner:
Original Code
NWF.FormFiller.Events.RegisterAfterReady(function () {
NWF$("#" + Specialty).on("change", function(event){
NWF$("#" + SelectAll).prop("checked", false);
});
NWF$("#" + SelectAll).on("change", function(event){
var isChecked = NWF$(event.target).prop("checked");
if (isChecked) {
NWF$("#" + emailID).siblings("table").find("input:checkbox").each(function(index, checkbox){
NWF$(checkbox).prop("checked", true);
});
}
});
});
Modification
NWF.FormFiller.Events.RegisterAfterReady(function(){
NWF$("#" + selectAll).on("change", function(event){
var isChecked = NWF$(event.target).prop("checked");
if (isChecked) {
NWF$("#" + mediaQualityChecks).siblings("table").find("input:checkbox").each(function(index, checkbox){
NWF$(checkbox).prop("checked", true);
}
}
});
});
- Removed the top portion of the function (event), which may be why it didn't work... as I'm assuming with my cursory knowledge that since I removed it, the function (event) is no longer defined.
Javascript IDs
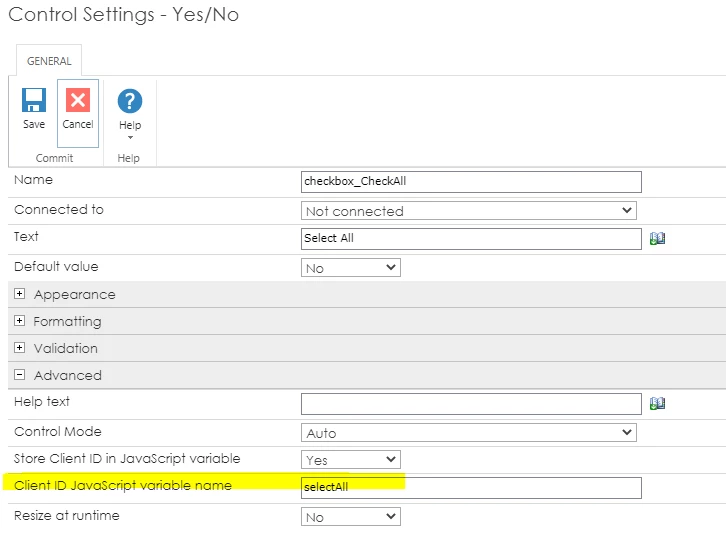
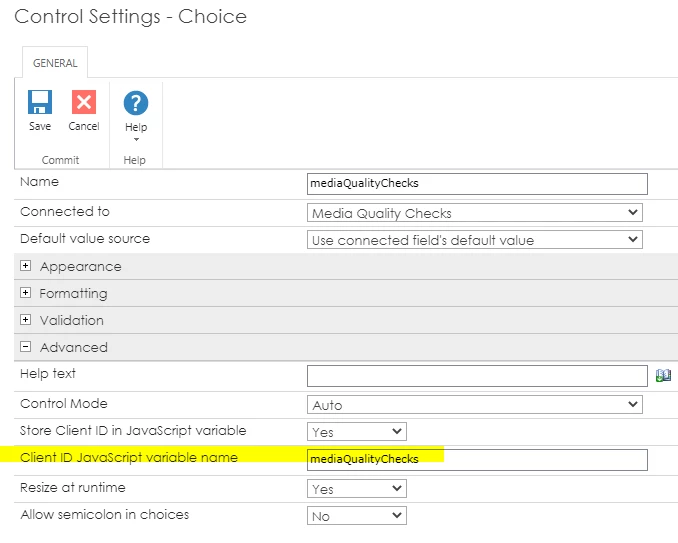
Thanks for any clarity!
I see.
Let's say that I have the following form with the same original Set All Yes/No checkbox, and a Choice Control with some amount of options:
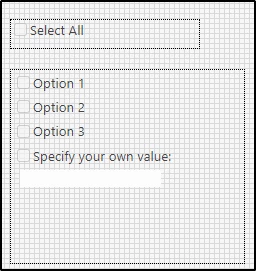
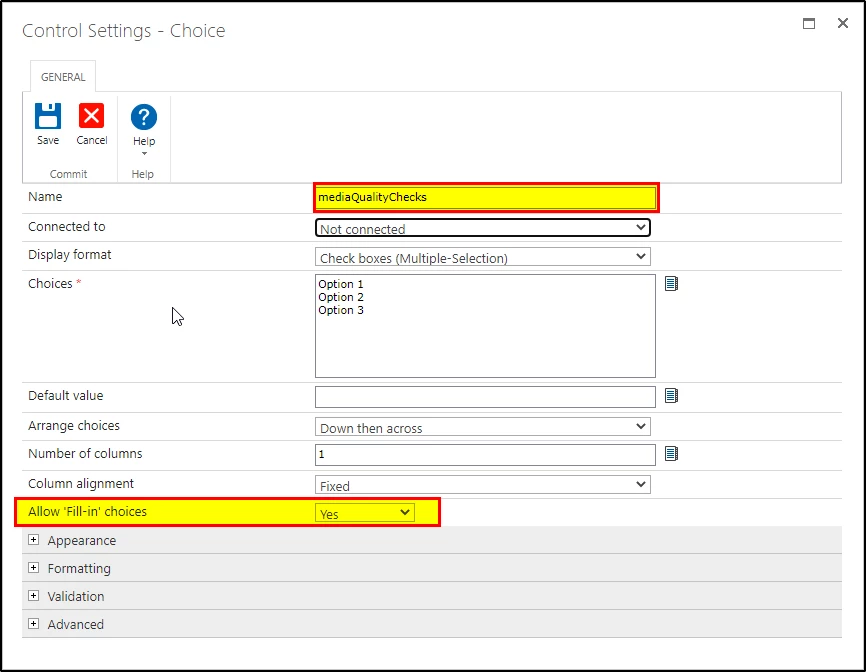
Dropping the following code into the Custom JavaScript dropdown of our Form's Settings:
NWF.FormFiller.Events.RegisterBeforeReady(function () {
var selectAllControlName = "control_SelectAll";
var targetChoiceControlName = "mediaQualityChecks";
var includeFillIn = true;
var triggerUpdate = true;
var resetToggle = false;
NWF$("[data-controlname='" + selectAllControlName + "']").on("change", function(event ){
var currentContext = NWF.FormFiller.Functions.GetParentContext(NWF$(event.target));
var choiceControl = currentContext.find("[data-controlname='" + targetChoiceControlName + "']");
var choices = choiceControl.find("input[type='checkbox'][value!='**Fillin**']");
var fillinChoice = choiceControl.find("input[type='checkbox'][value='**Fillin**']");
var selectAllChecked = event.target.checked;
var needsUpdate = false;
if (selectAllChecked) {
choices.each(function(i, choice){
if (!choice.checked) {
choice.checked = true;
if (!needsUpdate) {
needsUpdate = true;
}
}
});
if (includeFillIn && fillinChoice.prop("checked") === false) {
fillinChoice.prop("checked", true);
if (!needsUpdate) {
needsUpdate = true;
}
}
if (resetToggle) {
event.target.checked = false;
}
if (needsUpdate && triggerUpdate) {
choiceControl.find(".nf-associated-control input").first().trigger("change");
}
}
});
});
Should produce the following behavior:
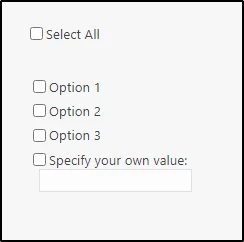
When Select All has been checked:
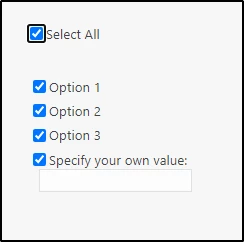
The code has several changes from my previous answer and now has a few more user settable options:
var selectAllControlName = "control_SelectAll";
var targetChoiceControlName = "mediaQualityChecks";
var includeFillIn = true;
var triggerUpdate = true;
var resetToggle = false;
While you should likely only care about setting the values of control name variables (assuming your control names are different or you want to use it somewhere else in a different form), the other options are there to help you fine tune things.
Here is all of the code with comments so that you can better understand what each line is doing:
/* Choice Control Target Version */
NWF.FormFiller.Events.RegisterBeforeReady(function () {
/*
The Control Name of your Select All Toggle Control
*/
var selectAllControlName = "control_SelectAll";
/*
The Control Name of your Choice Control
*/
var targetChoiceControlName = "mediaQualityChecks";
/*
Option to set whether this code should include a Fill In checkbox
*/
var includeFillIn = true;
/*
Option to set whether we should force an update to the control
so that any dependent form formulas also update once we are finished
*/
var triggerUpdate = true;
/*
Option to set the SelectAll checkbox back to unchecked afterwards
*/
var resetToggle = false;
/* Set up an Event Handler whenever the SelectAll checkbox has been changed */
NWF$("[data-controlname='" + selectAllControlName + "']").on("change", function(event ){
/* Get the Control's current form context */
var currentContext = NWF.FormFiller.Functions.GetParentContext(NWF$(event.target));
/* Find the Choice Control with the checkboxes that need checking */
var choiceControl = currentContext.find("[data-controlname='" + targetChoiceControlName + "']");
/* Get all of the checkboxes EXCEPT the FillIn */
var choices = choiceControl.find("input[type='checkbox'][value!='**Fillin**']");
/* Get ONLY the FillIn checkbox */
var fillinChoice = choiceControl.find("input[type='checkbox'][value='**Fillin**']");
/* Determine if this event was fired because of SelectAll being checked */
var selectAllChecked = event.target.checked;
/*
Create a flag that lets us know we made changes to the Choice Control.
We want to know if our code actually checked any of the boxes!
*/
var needsUpdate = false;
/* If the SelectAll was checked by the user */
if (selectAllChecked) {
/* Iterate through each choice */
choices.each(function(i, choice){
/* If the choice isn't checked... */
if (!choice.checked) {
/* Check it! */
choice.checked = true;
/*
Now we know that we've updated the choice control
in some meaningful way. So, if the 'needsUpdate'
flag is still set to false...
*/
if (!needsUpdate) {
/* Set it to true! */
needsUpdate = true;
}
}
});
/*
If the option to include the FillIn control was set to true,
AND there actually IS an unchecked FillIn Control...
*/
if (includeFillIn && fillinChoice.prop("checked") === false) {
/* Check it! */
fillinChoice.prop("checked", true);
/*
Once again we know that we've changed the Choice Control
in some meaningful way. So, if the 'needsUpdate' hasn't
already been set to true, we should do it now!
*/
if (!needsUpdate) {
needsUpdate = true;
}
}
/*
If the resetToggle is set to true, and you want the SelectAll
control to return to an unchecked state...
*/
if (resetToggle) {
/* SelectAll is unchecked */
event.target.checked = false;
}
/*
Lastly, if we know the Choice Control was changed and needs to
broadcast those changes to any Rules or Calculated Controls
AND the 'triggerUpdate' flag is set to true, then...
*/
if (needsUpdate && triggerUpdate) {
/*
We find the first child input to our Choice Control's
inner div with the '.nf-associated-control' class on it,
and proceed to trigger the change event.
*/
choiceControl.find(".nf-associated-control input").first().trigger("change");
}
}
});
});
By default this code should work inside or outside of a Repeating Section, and will (if you leave the triggerUpdate variable set to true) update the Control so that it informs any dependencies (Formatting / Validating Rules, or Calculated Controls) that they should update.
We can see this if we add a Calculated Control to the form and set its value to equal our Choice Control:
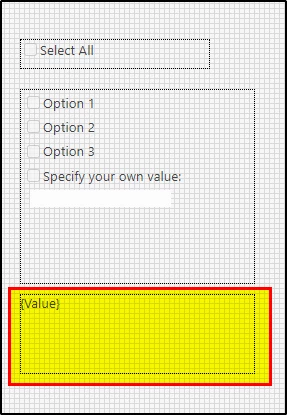
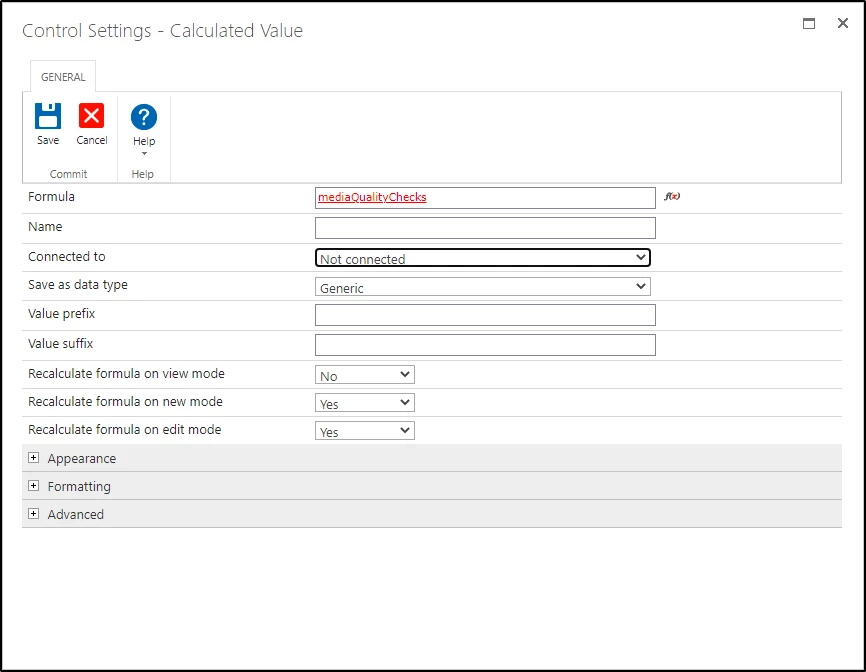
Now when we toggle the Select All, the Calculated Control's value is updated to match our Choice Controls:
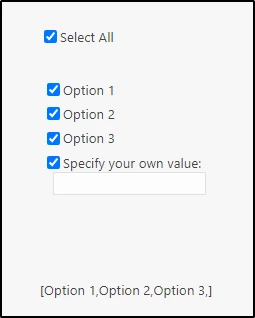
(if you had a value in the fillin)
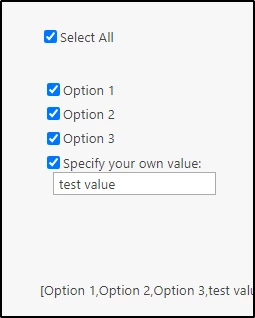
let me know if this works better for you.
Thank you so much for your time in writing the code and commentating it!!!
This works like a charm.