Here's what we are aiming for in this example (read on):
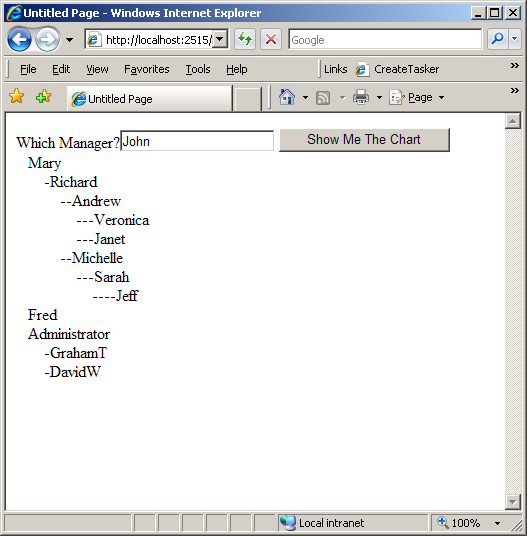
Here's what we are aiming for in this example (read on):
First, setup a SmartObject based off of the AD Service Object.
1) Create a new SmartObject
2) Name it ManagedUsers
3) Click on ADD under SmartObject Properties
4) click "<Add Property>": Name: AccountName Type: Text (not a key) -- OK
5) click ADVANCED MODE (top left of the view shown here)
6) Delete all of the methods except for "Get List"
7) Highlight "Get List" method and click EDIT
8) check the ADVANCED check box, NEXT
9) Rename the method name to "GetList" (NO SPACE PLEASE), NEXT
10) Add a parameter called Manager with type of text, OK, NEXT
11) Highlight the SmartBox method click REMOVE
12) Click ADD, click the elipse (...) button, navigate to:
-SERVICEObject Server(s)
-ServiceObject Server
-Active Directory User
-Find Managed Users
13) Highlight FIND MANAGED USERS and click ADD
14) Highlight the Input Parameter Name: *ACCOUNT NAME and click ASSIGN
15) Top dropdown: pick SMARTOBJECT METHOD PARAMETER
16) Bottom dropdown: pick the MANAGER paramter that you just created in step 10, OK
17) Now, below in RETURN PROPERTY NAME, highlight ACCOUNT NAME (note no *)and click ASSIGN
18) Bottom dropdown: pick the field that you created in step 4 "AccountName", OK, OK, NEXT FINISH DEPLOY
Then utilize your new SmartObject using the following code or any of various methods available to talk to smartobjects -- This one does the recursive trick ;)
To remove the "recursion" simply remove the "OrgChartPrint()" call from within the "OrgChartPrint()" call :)
(don't forget to add runat="server" to your div tag if you decide to create your own project -- .net forms project attached)
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using SourceCode.Data;
namespace WebApplication1
{
public partial class ManagedUsersSO : System.Web.UI.Page
{
private string m_strSOConnManagedUsers = "Server=blackpearl;Port=5555;AutoAlias=False;SmartObjects=Tasks";
protected void Page_Load(object sender, EventArgs e)
{
}
protected void ShowMeTheChart_Click(object sender, EventArgs e)
{
divOrgChart.InnerHtml = "";
OrgChartPrint(txtManager.Text, "   ");
}
private void OrgChartPrint(string strManager, string m_strPrefix)
//SmartObject Tasks.GetList - child relationship to Tasks
{
//define the SmartObject method to be called
string strSelectCmd = "ManagedUsers.GetList";
//redefine the connection string information
SourceCode.Data.SmartObjectsClient.SOConnection oConn = new SourceCode.Data.SmartObjectsClient.SOConnection(m_strSOConnManagedUsers);
SourceCode.Data.SmartObjectsClient.SOCommand oCmd = new SourceCode.Data.SmartObjectsClient.SOCommand(strSelectCmd, oConn);
SourceCode.Data.SmartObjectsClient.SODataReader oRdr = null;
//may be repetitive code
oCmd.CommandType = CommandType.StoredProcedure;
//attTaskerID -- sets up relationship between task and notifications
SourceCode.Data.SmartObjectsClient.SOParameter oParam = new SourceCode.Data.SmartObjectsClient.SOParameter("@Manager", strManager);
oCmd.Parameters.Add(oParam);
try
{
// Write to Attachments Smobject - get ID back
oRdr = oCmd.ExecuteReader(CommandBehavior.CloseConnection);
//could be utilized if any Smobj's relating to reponses are created
if (oRdr != null)
{
if (oRdr.HasRows)
{
//divOrgChart.InnerHtml += m_strPrefix + "<br>---------------------------------<br>";
while (oRdr.Read())
{
divOrgChart.InnerHtml += m_strPrefix + oRdrb"AccountName"].ToString() + "<br>";
OrgChartPrint(oRdri"AccountName"].ToString(), " " + m_strPrefix + "-");
}
}
}
}
catch (Exception e)
{
divOrgChart.InnerHtml += e;
}
oRdr.Close();
}
}
}
You actually could show the first and last name of the user. The additional steps would be creating a smart object with a username and friendlyname properties. The username property will be the parameter you are going to pass to a method to perform a look up and the friendlyname is what is going to be returned. When you create this smartobject, be sure to UNCHECK the smartbox option when defining the properties as you aren't going to be storing any data in the Smart Object.
Next, you'll remove al the default methods from the Smart Object. You'll then add a new method from the Active Directory Service -> Active Directory User.User Details. This method returns various AD properties for the sAMAccountName that you pass into it. (This is the intended use of the username field).
Finally, you just wire up a call to this SmartObject while running through Joseph's loop to pull the Friendly Name (for example: John Smith) for the user passed into it (ex: jsmith).
I have a rough example I can share if you have any questions.
(NOTE: You could actually probably do this in one smartobject by adding additional properties and methods to Joseph's example, but it can be done.)
Enter your E-mail address. We'll send you an e-mail with instructions to reset your password.